An editing toolbar for alexwlchan.net
I recently wrote a small bookmarklet that gives me an editing toolbar for this site:
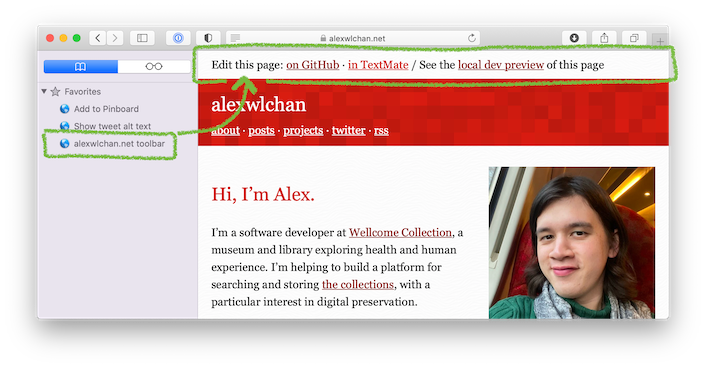
When I run the bookmarklet, it adds a toolbar to the top of the page I’m looking at, which lets me do various useful things:
- Open the page for editing on GitHub
- Open the page for editing in my text editor in a local checkout
- Toggle between the local preview and live versions of the site
I can’t imagine anybody else wants this particular bookmarklet, but I’ll explain how it works in case anybody else wants to build something similar.
First, I added the following HTML to one of my templates:
<meta name="page-source-path" content="{{ page.path }}">
This is a Jekyll page variable that contains the path to the source code for a page. For example, on this page this renders as:
<meta name="page-source-path" content="_posts/2021/2021-09-28-editing-toolbar.md">
Adding it to the template means it gets baked into every page, and I can read the value with JavaScript.
Then I have some JavaScript which reads the value, and uses it to insert the appropriate blob of HTML at the top of the page:
var sourcePath = document.querySelector("meta[name=page-source-path]").attributes["content"].value;
/* I run the site on http://localhost:5757 when working locally, so that's
* the URL I want to switch to for the dev preview. */
if (document.location.href.startsWith("https://alexwlchan.net/")) {
var altEnvironment = "local dev preview";
var altUrl = document.location.href.replace("https://alexwlchan.net/", "http://localhost:5757/");
} else {
var altEnvironment = "live version";
var altUrl = document.location.href.replace("http://localhost:5757/", "https://alexwlchan.net/");
}
/* Are we running on iOS? I don't have a local checkout of the repo on iOS
* and I don't run the localhost version of the site, so adding those links
* isn't useful. On iOS, the only link I want is to the GitHub source, so I
* can do quick edits in the browser.
*
* See https://stackoverflow.com/q/9038625/1558022 */
var iOS = [
'iPad Simulator',
'iPhone Simulator',
'iPod Simulator',
'iPad',
'iPhone',
'iPod'
].includes(navigator.platform) || (navigator.userAgent.includes("Mac") && "ontouchend" in document);
if (iOS) {
document.querySelector("body").innerHTML = `
<article style="padding-bottom: 8px; padding-top: 8px;">
Edit this page:
<ul class="dot_list" style="display: inline-block; margin: 0;">
<li><a href="https://github.com/alexwlchan/alexwlchan.net/blob/live/src/${sourcePath}">on GitHub</a></li>
</ul>
</article>
` + document.querySelector("body").innerHTML;
} else {
/* The link to open in my text editor uses a txmt:// URL, which is the URL
* scheme for opening files in TextMate, my editor of choice. */
document.querySelector("body").innerHTML = `
<article style="padding-bottom: 8px; padding-top: 8px;">
Edit this page:
<ul class="dot_list" style="display: inline-block; margin: 0;">
<li><a href="https://github.com/alexwlchan/alexwlchan.net/blob/live/src/${sourcePath}">on GitHub</a></li>
<li><a href="txmt://open?url=file://~/repos/alexwlchan.net/src/${sourcePath}">in TextMate</a></li>
</ul>
/
See the <a href="${altUrl}">${altEnvironment}</a> of this page
</article>
` + document.querySelector("body").innerHTML;
}
Fun fact: this is my first time using JavaScript template literals, which are a super nice feature I wish I’d known about before. I’m only about five years behind the cutting edge of web development!
I used Peter Coles’s bookmarklet creator to turn this into my bookmarklet, and now I have an editing toolbar I can get whenever I need it. It’s been particularly useful when I want to make edits on my phone – I can jump from a page to the source code in GitHub, make a tweak in GitHub’s editor, and my continuous deployment pipeline will publish the updated version shortly afterwards.
I can’t imagine anyone else wants this exact bookmarklet, but if you have your own static site then something like this might be useful.